Intent
The Singleton design pattern is a creational design pattern that ensures there is only one instance of a class throughout the lifecycle of the application to provide single global access to the object.
Motivation
At times it becomes significantly important that there is only one instance of some classes that may exist within the application. The question arises Why do we need to control the number of instances of a class? Think in terms of sharing a common resource for example a database connection, a service, a file system, logging, driver objects, caching, or a repository that manages different entities. We can ensure that there should be only a single instance of such an object through the use of a global access point by defining a variable and providing controlled access to this variable.
Applicability
- There should be exactly a single instance of a class accessible to the clients using a global access point.
- The class should manage this access point and maintain a single instance of itself.
Consequences
- It controls access to a single instance of a given class.
- It maintains a single instance responsible for sharing common resources.
- The class that implements the Singleton Design Pattern manages its own instance inside it and provides a global access point.
Implementation Strategy
- The implementation class defines a private constructor to prevent its invocation.
- The Singleton class declares a static instance field to maintain a single instance of itself.
- The Singleton class provides a static method as a global entry or access point to this single instance variable of itself.
Class Diagram
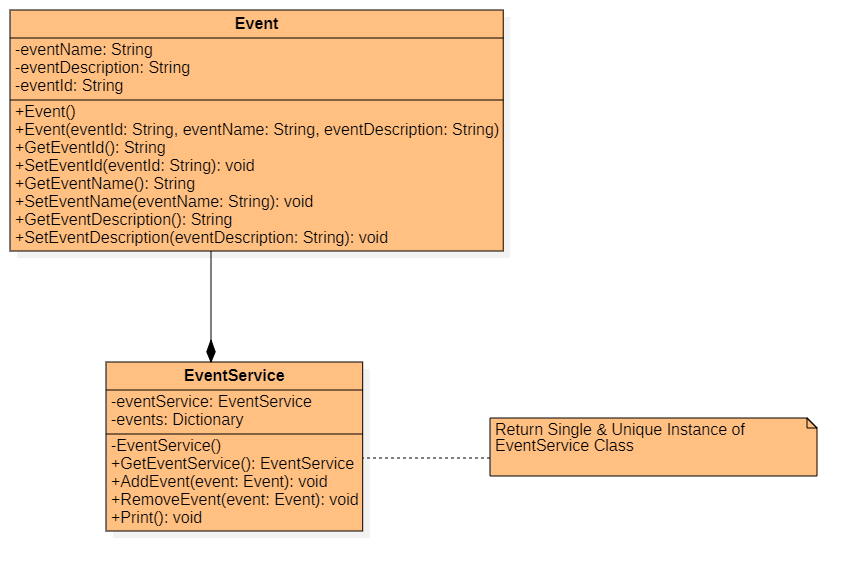
Source Code
Event.cs
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace SingletonDesignPatternApp
{
/// <summary>
/// Simple entity class to represent an Event
///
/// Programmer: CodingHelpLine
/// Web: https://codinghelpline.org
/// </summary>
internal class Event
{
// Properties
public string EventId { get; set; }
public string EventName { get; set; }
public string EventDescription { get; set; }
}
}
EventService.cs
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace SingletonDesignPatternApp
{
/// <summary>
/// Class to implement the Singleton Design Pattern and provides a mechanism
/// to maintain a single instance of itself.
///
/// Programmer: CodingHelpLine
/// Web: https://codinghelpline.org
/// </summary>
internal class EventService
{
// Two things
// 1. collection to store events
// 2. Instance of this class
private Dictionary<String, Event> events;
// Static reference to current class
private static EventService instance;
/// <summary>
/// Private constructor to limit creation of instances.
/// </summary>
private EventService()
{
events = new Dictionary<String, Event>();
instance = null;
}
/// <summary>
/// Function to maintain or provide Global Access Point to the instance
/// this class.
///
/// </summary>
/// <returns></returns>
public static EventService GetInstance()
{
// Check if the instance is null
if(instance == null)
{
instance = new EventService();
}
return instance;
}
/// <summary>
/// Function add new Event into the repository
/// </summary>
/// <param name="eventObject"></param>
public void AddEvent(Event eventObject)
{
this.events.Add(eventObject.EventId, eventObject);
}
/// <summary>
/// Remove event from the Dictionary.
/// </summary>
/// <param name="eventgId"></param>
public void RemoveEvent(string eventgId)
{
this.events.Remove(eventgId);
}
/// <summary>
/// Print the events
/// </summary>
public void Print()
{
foreach (var evt in events)
{
Event e = evt.Value;
Console.WriteLine($"({e.EventId}, {e.EventName}, {e.EventDescription}");
}
}
}
}
Program.cs
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace SingletonDesignPatternApp
{
internal class Program
{
/// <summary>
/// Client Code to test the Functionality of Singleton Design Pattern.
///
/// Programmer: CodingHelpLine
/// Web: https://codinghelpline.org
/// </summary>
/// <param name="args"></param>
static void Main(string[] args)
{
// Create singleton object
EventService service = EventService.GetInstance();
Event e1 = new Event();
e1.EventId = "E1";
e1.EventName = "Dinner";
e1.EventDescription = "Family Dinner";
Event e2 = new Event();
e2.EventId = "Ee";
e2.EventName = "Meeting";
e2.EventDescription = "Business Meeting";
// Add elements
service.AddEvent(e1);
service.AddEvent(e2);
service.Print();
// Remove one element
service.RemoveEvent("E1");
// Print again
Console.WriteLine("Event Servics: ");
service.Print();
// Create new Instance
EventService svc2 = EventService.GetInstance();
Console.WriteLine("\n\nSecond Instance of Event Service:");
svc2.Print();
Console.ReadKey();
}
}
}